Assignments » File Handling » Set 3
File Handling - Binary File
[Set – 3]
1. Write a Python program that manages employee records using a binary file. The program should have the following functions:
add_employee()
This function should prompt the user to input data for an employee, including Employee ID, Name, Department, and Salary. The data should be stored in a dictionary format: {'ID': ID, 'Name': Name, 'Department': Department, 'Salary': Salary}. The function should then add the dictionary object to the emp.dat binary file using pickling.
display_records()
This function should display all the records of employees stored in emp.dat file on the screen. Each employee's details should be displayed with their corresponding attributes: Employee ID, Name, Department, and Salary.
search_employee(id)
This function should receive an Employee ID as an argument. It should search through the emp.dat file and display the details of the employee with the given ID. If no employee with the provided ID is found, display an appropriate message.
In the program, include a menu-based interface that allows users to choose which function to execute.
Sample Output
Employee Record Management System
Menu:
1. Add Employee
2. Display Employee Records
3. Search Employee
4. Exit
Enter your choice: 1
Enter Employee ID: 101
Enter Employee Name: Rajesh Kumar
Enter Employee Department: IT
Enter Employee Salary: 60000
Employee added successfully.
Menu:
1. Add Employee
2. Display Employee Records
3. Search Employee
4. Exit
Enter your choice: 2
Employee Records:
Employee ID: 101
Name: Rajesh Kumar
Department: IT
Salary: 60000
-----------------------------
Menu:
1. Add Employee
2. Display Employee Records
3. Search Employee
4. Exit
Enter your choice: 3
Enter Employee ID to search: 101
Employee found:
Employee ID: 101
Name: Rajesh Kumar
Department: IT
Salary: 60000
Menu:
1. Add Employee
2. Display Employee Records
3. Search Employee
4. Exit
Enter your choice: 4
Thanks for using Employee Record Management System
Solution
import pickle
def add_employee():
emp_id = input("Enter Employee ID: ")
emp_name = input("Enter Employee Name: ")
emp_department = input("Enter Employee Department: ")
emp_salary = input("Enter Employee Salary: ")
employee = {}
employee['ID']= emp_id
employee['Name']= emp_name
employee['Department']= emp_department
employee['Salary']= emp_salary
file = open('emp.dat', 'ab')
pickle.dump(employee, file)
file.close()
print("Employee added successfully.")
def display_records():
file = open('emp.dat', 'rb')
try:
while True:
employee = pickle.load(file)
print("\nEmployee Records:")
print("Employee ID:", employee['ID'])
print("Name:", employee['Name'])
print("Department:", employee['Department'])
print("Salary:", employee['Salary'])
print("-----------------------------")
except EOFError:
pass
file.close()
def search_employee(emp_id):
try:
file = open('emp.dat', 'rb')
while True:
employee = pickle.load(file)
if employee['ID'] == emp_id:
print("\nEmployee found:")
print("Employee ID:", employee['ID'])
print("Name:", employee['Name'])
print("Department:", employee['Department'])
print("Salary:", employee['Salary'])
file.close()
return
except EOFError:
print("Employee with ID", emp_id, "not found.")
finally:
file.close()
def main():
print("Employee Record Management System\n")
while True:
print("\nMenu:")
print("1. Add Employee")
print("2. Display Employee Records")
print("3. Search Employee")
print("4. Exit")
choice = input("\nEnter your choice: ")
if choice == '1':
add_employee()
elif choice == '2':
display_records()
elif choice == '3':
emp_id = input("\nEnter Employee ID to search: ")
search_employee(emp_id)
elif choice == '4':
print("Thanks for using Employee Record Management System")
break
else:
print("Invalid choice. Please select a valid option.")
main()
PyForSchool's Computer Science with Python Class XII 2023-24 [Revision Notes & Question Bank]
An outstanding 4.6 out of 5-star rating on Amazon! 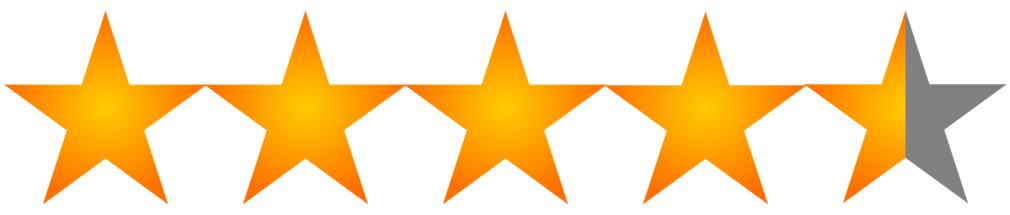
Buy Now
2. Write a Python program that manages book records using a binary file called book.dat. The program should have the following functions:
add_record()
This function should prompt the user to input data for a book, including the Book Number, Book Name, Author, and Price. The data should be stored in a list format: [BookNo, Book_Name, Author, Price]. The function should then add the book data to the book.dat binary file using pickling.
display_records()
This function should display all the records stored in the book.dat file on the screen. Each record should be displayed with its corresponding attributes: Book Number, Book Name, Author, and Price.
books_by_author()
This function should ask the user to input an author's name. It should then search through the records in the book.dat file and display all the records for books written by the given author.
books_by_price(price)
This function should receive a maximum price as an argument. It should search through the records in the book.dat file and display all the records for books with a price less than the provided maximum price.
copy_data()
This function should read the contents of the book.dat file. It should copy the records of books whose price is more than $500 to a new file named costly_book.dat using pickling. The function should return the count of records copied.
delete_record(book_number)
This function should receive book number as an argument.. If a record with the given book number exists, it should be deleted from the book.dat file. If the record does not exist, display an error message.
update_record(book_number)
This function should receive a maximum price as an argument. If a record with the given book number exists, prompt the user to update the Book Name, Author, and Price for that record. If the record does not exist, display an error message.
In the program, include a menu-based interface that allows users to choose which function to execute.
Sample Output
Book Record Management System
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 1
Enter Book Number: 123
Enter Book Name: The Great Indian Novel
Enter Author: Arundhati Roy
Enter Price: 450.50
Book record added successfully.
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 1
Enter Book Number: 234
Enter Book Name: Midnight's Children
Enter Author: Salman Rushdie
Enter Price: 550.75
Book record added successfully.
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 2
Book Number: 123
Book Name: The Great Indian Novel
Author: Arundhati Roy
Price: 450.5
-----------------------------
Book Number: 234
Book Name: Midnight's Children
Author: Salman Rushdie
Price: 550.75
-----------------------------
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 3
Enter Author's Name: Arundhati Roy
Book Number: 123
Book Name: The Great Indian Novel
Author: Arundhati Roy
Price: 450.5
-----------------------------
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 4
Enter Maximum Price: 500
Book Number: 123
Book Name: The Great Indian Novel
Author: Arundhati Roy
Price: 450.5
-----------------------------
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 5
2 records copied to costly_book.dat
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 6
Enter Book Number to delete: 234
Record deleted successfully.
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 7
Enter Book Number to update: 123
Current Book Name: The Great Indian Novel
Enter New Book Name: The Palace of Illusions
Current Author: Arundhati Roy
Enter New Author: Chitra Banerjee Divakaruni
Current Price: 450.5
Enter New Price: 420.25
Record updated successfully.
Menu:
1. Add Record
2. Display Records
3. Books by Author
4. Books by Price
5. Copy Costly Books
6. Delete Record
7. Update Record
8. Exit
Enter your choice: 8
Thanks for using Book Record Management System
Solution
import pickle
import os
def add_record():
book_number = input("Enter Book Number: ")
book_name = input("Enter Book Name: ")
author = input("Enter Author: ")
price = float(input("Enter Price: "))
book_data = [book_number, book_name, author, price]
file = open('book.dat', 'ab')
pickle.dump(book_data, file)
file.close()
print("Book record added successfully.")
def display_records():
file = open('book.dat', 'rb')
try:
while True:
book_data = pickle.load(file)
print("\nBook Number:", book_data[0])
print("Book Name:", book_data[1])
print("Author:", book_data[2])
print("Price:", book_data[3])
print("-----------------------------")
except EOFError:
pass
file.close()
def books_by_author():
author = input("Enter Author's Name: ")
found = False
file = open('book.dat', 'rb')
try:
while True:
book_data = pickle.load(file)
if book_data[2] == author:
print("\nBook Number:", book_data[0])
print("Book Name:", book_data[1])
print("Author:", book_data[2])
print("Price:", book_data[3])
print("-----------------------------")
found = True
except EOFError:
pass
file.close()
if not found:
print("No records found for author:", author)
def books_by_price(max_price):
found = False
file = open('book.dat', 'rb')
try:
while True:
book_data = pickle.load(file)
if book_data[3] < max_price:
print("\nBook Number:", book_data[0])
print("Book Name:", book_data[1])
print("Author:", book_data[2])
print("Price:", book_data[3])
print("-----------------------------")
found = True
except EOFError:
pass
file.close()
if not found:
print("No records found for price less than:", max_price)
def copy_data():
count = 0
src_file = open('book.dat', 'rb')
dest_file = open('costly_book.dat', 'wb')
try:
while True:
book_data = pickle.load(src_file)
if book_data[3] > 500:
pickle.dump(book_data, dest_file)
count += 1
except EOFError:
pass
src_file.close()
dest_file.close()
return count
def delete_record(book_number):
src_file = open('book.dat', 'rb')
dest_file = open('temp.dat', 'wb')
found = False
try:
while True:
book_data = pickle.load(src_file)
if book_data[0] == book_number:
found = True
else:
pickle.dump(book_data, dest_file)
except EOFError:
pass
src_file.close()
dest_file.close()
if not found:
print("No record found for book number:", book_number)
else:
print("Record deleted successfully.")
os.remove('book.dat')
os.rename('temp.dat', 'book.dat')
def update_record(book_number):
src_file = open('book.dat', 'rb')
dest_file = open('temp.dat', 'wb')
found = False
try:
while True:
book_data = pickle.load(src_file)
if book_data[0] == book_number:
found = True
print("Current Book Name:", book_data[1])
new_book_name = input("Enter New Book Name: ")
print("Current Author:", book_data[2])
new_author = input("Enter New Author: ")
print("Current Price:", book_data[3])
new_price = float(input("Enter New Price: "))
book_data[1] = new_book_name
book_data[2] = new_author
book_data[3] = new_price
pickle.dump(book_data, dest_file)
except EOFError:
pass
src_file.close()
dest_file.close()
if not found:
print("No record found for book number:", book_number)
else:
print("Record updated successfully.")
os.remove('book.dat')
os.rename('temp.dat', 'book.dat')
def main():
print("Book Record Management System\n")
while True:
print("Menu:")
print("1. Add Record")
print("2. Display Records")
print("3. Books by Author")
print("4. Books by Price")
print("5. Copy Costly Books")
print("6. Delete Record")
print("7. Update Record")
print("8. Exit")
choice = input("\nEnter your choice: ")
if choice == '1':
add_record()
elif choice == '2':
display_records()
elif choice == '3':
books_by_author()
elif choice == '4':
max_price = float(input("Enter Maximum Price: "))
books_by_price(max_price)
elif choice == '5':
count = copy_data()
print(count, "records copied to costly_book.dat")
elif choice == '6':
book_number = input("Enter Book Number to delete: ")
delete_record(book_number)
elif choice == '7':
book_number = input("Enter Book Number to update: ")
update_record(book_number)
elif choice == '8':
print("Thanks for using Book Record Management System")
break
else:
print("Invalid choice. Please select a valid option.")
main()